Defining a Macro
Using a Macro
Repetition In Macros
Defining a Macro
In Rust macros declarations start from macro_rules like macro_rules! some_macro{() => ()}
. Here some_macro is name of the macro.
Example
macro_rules! add{ ($x:expr,$y:expr) => ($x + $y); }
Using a Macro
To use a Macro in rust we write it's name with an exclamation point like add!
.
Let say we define a macro that takes two expressions (say x and y) and evaluates to x + y
on compiling.
Example
macro_rules! add{ ($x:expr,$y:expr) => ($x + $y); } fn main(){ println!("{}",add!(3,5)); println!("{}",add!(3,add!(4,5))); println!("{}",add!({3-5},{5*3})); }
Output for the above example will be
8 12 13
So println!("{}",add!(3,add!(4,5)));
will be converted to
println("{}",3 + 4 + 5);
on compiling
Repetition In Macros
vec!
macro can take different no of parameters like let x = vec![1,2,3,4];
We can also create macros that can work with repeated values.
Example
macro_rules! double_vec{ ($($x:expr),*) => ({ let mut temp = Vec::new(); $( temp.push($x*2); )* temp }); } fn main(){ let new_vec = double_vec![2,3,5,6]; println!("{:?}",new_vec); }
Breaking Down the code, $(...)* means zero or more parameters $(...)+ means one or more parameters.
Here macro matches the pattern of parameters seperated by ',' and push twice the value of parameters in a vector and returns it.
Output for the above example will be
[4, 6, 10, 12]
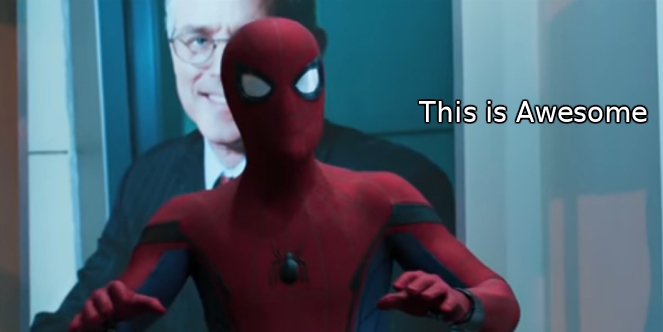
For Full Documentation Go To
Macros - Rust Documentation